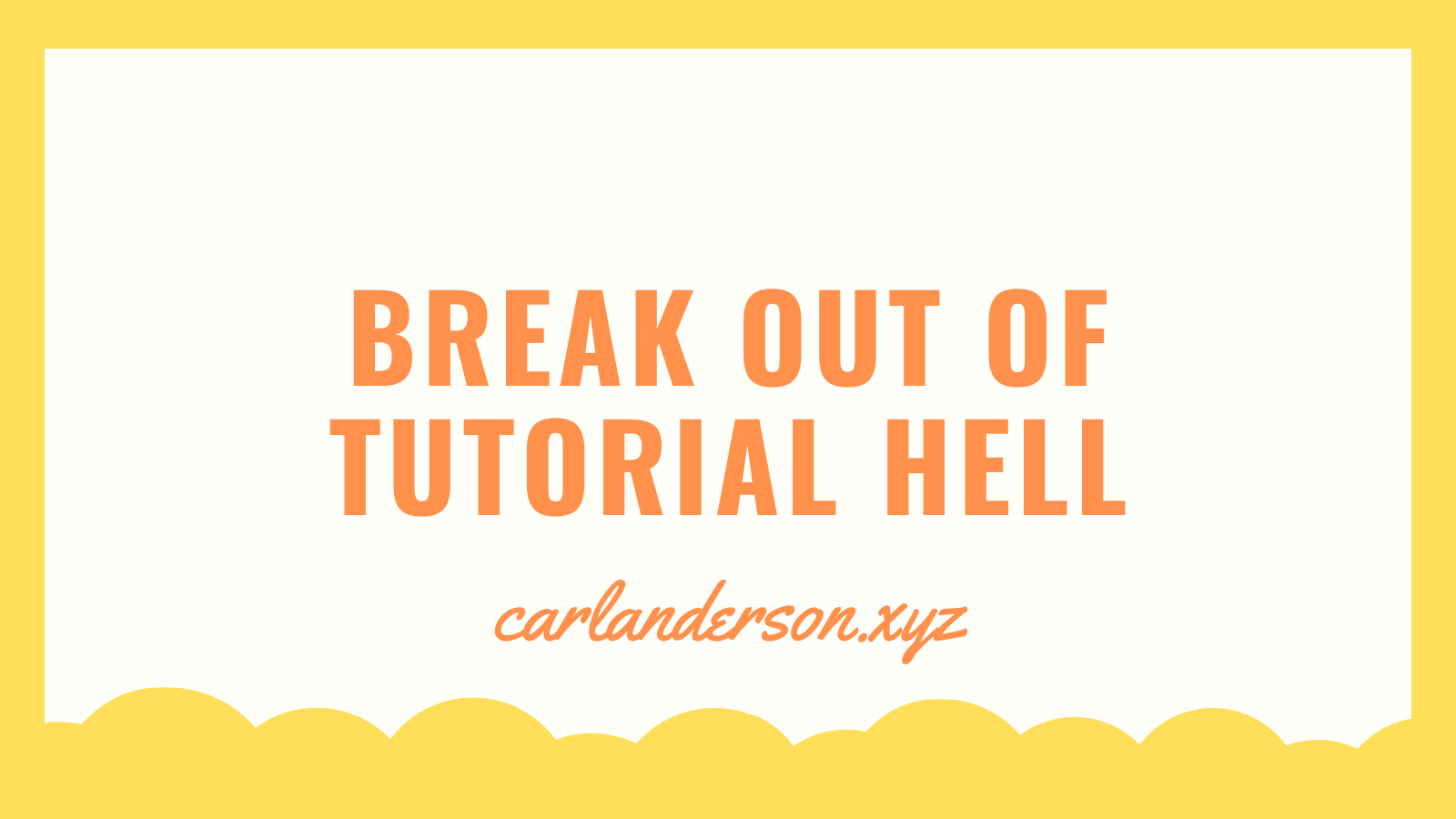
Break out of Tutorial Hell - What to Do When You're Going Nowhere
Insanity and tutorial hell is endlessly repeating courses and expecting something different. It doesn't work. Find out how to break the cycle.
Insanity and tutorial hell is endlessly repeating courses and expecting something different. It doesn't work. Find out how to break the cycle.
JavaScript
Creating a smooth expand/collapse animation seems easy. Surely you can set a transition on height: auto, and it'll just work? Sadly, the CSS gods aren't so kind. Instead of the smooth open and close you wanted, your element flashes to its new height. No smooth animation. As it turns
Basics
If you've ever tried to modify your inline CSS from JavaScript, you'll notice it can be quite verbose. // Don't do this const button = document.querySelector('#button'); button.addEventListener('click', () => { button.style.border = '1px solid blue'; button.style.borderRadius = '2px'; button.style.color = 'white'; // and so it goes }); It's dirty,
Have you ever added a button to a page with JavaScript expecting it to function fully, but when you clicked it nothing happened? You've double checked your code - you're creating the event listeners, the selectors are correct, but it's still not working! Chances are, if you've hit this situation,
Closures, as well as being a favourite interview question, are one of the more confusing parts of JavaScript. Despite the wealth of articles offering explanations of closures, there are dozens of threads on reddit and other forums asking for more help with closures. It's not hard to see why, since
Here's the question: What's the difference between these two methods of creating a function? function a() { //This is known as a function declaration console.log("Hello"); } var b = function() { //This is known as a function expression console.log("World"); } Both of these methods do almost the same thing, however there
UPDATE: There is now an aspect-ratio CSS property which can be used to set the ratio between width and height. The best way to make a square is now aspect-ratio: 1 / 1 If you remember the days of floats and table layouts, display: flex is absolutely magical... until you want
When you start working in Javascript and building apps and webpages, you get used to running code based on inputs - someone pressed a button, and then your code gets to run. This is fine for most webpages, but what if you want to to make changes on the page