How to Write JavaScript When You Don’t Know Where to Begin
Have you ever tried a coding problem, but ended up staring at a blank editor? Maybe you tried to write a simple function, but didn't even know where to begin?
It's frustrating.
After all, you've spent months working to understand JavaScript's basics. You've learned all there is to know about variables, arrays and functions... but when it comes to coding on your own, you struggle to write a single line.
It's not that you don't know JavaScript -- You're fully capable of reading code. It's just when it comes time to putting pen to paper, something is missing.
How do you get past the blank editor?
Firstly, it's important that you don't get discouraged. Starting another course is not the answer. What you do need is a method for breaking down your ideas so that you know what code to write.
I've broken down the system that I use to solve coding problems, and provided a step-by-step guide on how to go from idea to code. I'll take you through an example using the Fibonacci sequence to show how it works in action.
How to break down problems
(N.B. I refer to "English" a lot here, but you should use whatever language you're most comfortable with)
A flaw in the way beginners try to approach coding is that they try to solve the problem in JavaScript. This seems like a stupid statement, so I'll say it another way: you need to solve the problem in English first.
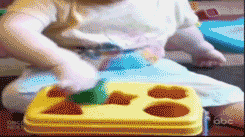
When we teach JavaScript, we show you the building blocks of the language. What we don't do is explain how to express ideas in JavaScript.
You need to express your ideas in English first, and then translate into JavaScript. This is done in the following four steps:
- Define your problem in English
- Define a solution in English
- Write your solution in pseudo-code
- Translate your solution to JavaScript
This still might not be very easy the first time you do it - it can take some getting used to. Spoken languages don't force you to clarify your ideas at the level required for coding.
As you do it more, you'll gain an understanding of what you can and can't do with code, and gain an innate sense for how to express your ideas in a way that translates easily.
Writing in Pseudo-code
Pseudo-code is an amalgamation of code and English. There's no correct way to write pseudo-code - you make it up as you go along. So long as it looks a little bit like code, you're doing well.
The reason we write pseudo-code is that it allows us to write in a language-agnostic way. We use it to skip over unnecessary details in a way that we can easily convert into code.
The beauty of pseudo-code over simply writing JavaScript is we can choose whatever level of detail we like. We can use "magic" statements to sketch our ideas without having to worry about implementation details. If it's helpful, we can even write pseudo-code in full sentences.
//Both of these functions are perfectly valid pseudo-code
printSortedArray(array):
sort array
print each item in array
printSortedArray(array):
// Bubble sort
while array is not sorted:
for each item in array:
if item+1 is less than item
swap item and item+1
for each item in array:
print item
Example: The Fibonacci sequence
I'm going to run through an example of breaking down a problem and writing pseudo-code. We're going to create a function which returns the nth value of the Fibonacci sequence.
There are two things to note throughout this process:
- It is highly personal. What I come up with may be different from you.
- It takes longer than it looks. This is a blog post - you skip straight to the end result. You don't get to see the time spent thinking about the problem.
Plain English
Our main goal into this step is to clarify everything. The more concrete and specific we can be, the better. Initially, it's a good idea to simply define everything, and then start stating facts about your definitions.
If we define the Fibonacci sequence problem:
- The Fibonacci sequence is the sequence
1, 1, 2, 3, 5, 8
etc. - To calculate any value of the Fibonacci sequence, I have to know the two previous values in the series.
- If I want to know a specific value in the sequence, I need to know every prior value in the chain.
This may not seem like much, but it does give us enough to define a simple solution:
- To get the value for
n
, I need to generate the entire Fibonacci sequence up ton
.
If you're able to (and the problem allows for it), you can think up multiple solutions, and choose one. If it doesn't work out, it's good to be able to come back and try a different path.
Pseudo-code
Alright. We now have a specific way to get the n
th value of the Fibonacci series: create all the numbers up until n. At this stage, we want to broadly think about how we're going to code this.
As I mentioned, the beauty of pseudo-code is that it lets me solve the problem at different levels of detail. It can often pay to solve the problem using "magic" the first time around and add detail as we need it.
This is how I'd devise a "magic" solution:
fibonacci (n):
loop between 0 and n
sum two previous fibonacci numbers
when n, return value
It isn't detailed enough to turn directly into code because of the magic "two previous fibonacci numbers", but the basic approach is solid. We could absolutely use this as a blueprint to start coding from, filling in the blanks as we go.
Since this is a demonstration, we're going to do one more round of pseudo-code to add some more detail.
In this round, we want a little less magic: How do we get the two previous Fibonacci numbers?
function fibonacci(n):
// We can't get n-2 for 0 and 1, so return them directly
if n is 0 or 1, return 1
// Set these to the first two numbers of the fibonacci sequence
prev1 = 1
prev2 = 1
// use 2 here because we've already done when n is 0 and 1
loop from 2 until n:
current = prev1 + prev2
// Update the previous numbers, so we're ready for the next loop
prev2 = prev1
prev1 = current
return current
At a glance, this looks completely different to our previous solution, but it's exactly the same. We've just clarified how we're going to store the previous Fibonacci values inside of variables.
This is now a complete solution. While there are some minor details that I may need to add when coding, it will translate into JavaScript almost 1:1.
JavaScript
This step should be self-explanatory. Take the pseudo-code code you have, and turn it into the final JavaScript.
You'll likely need to make some final clarifying decisions (like choosing to use <=
inside of the for loop), but this should look very similar to your pseudo-code.
function fibonacci(n) {
// We can't get n-2 for 0 and 1, so return them directly
if (n === 0 || n === 1) { return 1; }
let prev1 = 1;
let prev2 = 1;
let current;
// use 2 here because we've already done when n is 0 and 1
for (let i = 2; i <= n; i++) {
current = prev1 + prev2;
// Update the previous numbers, so we're ready for the next loop
prev2 = prev1;
prev1 = current;
}
return current;
}
This isn't the most concise solution to the Fibonacci sequence, but it is a perfectly valid one. It will definitely pass a coding interview.
As I said before, this may not come naturally to you at the beginning. The next step for you to take is to practice. You can go over to sites like HackerRank to try some of their coding problems, or you can start working on your own practice projects.
If you found this article helpful, be sure to sign up to my mailing list below.